-
- Chrome extensions.
- Many websites use your cpu without your consent to mine crypto. There are extensions that block this, but they become outdated pretty quickly.
- Added JSON formatter. Makes json responses look pretty.
- OctoLinker turns import/require/include statements into links (on github), so you can jump to other projects quickly.
- Octotree adds a muuuuuch better directory tree on the left sidebar of github.
- And Refined Github. So many improvements to github UI/UX.
- My current list:
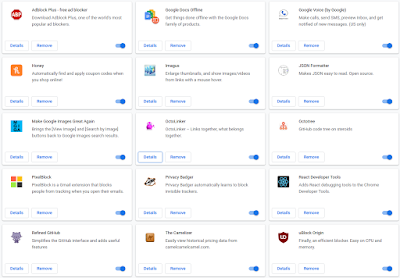
- Worked on bmahlstedt.com more.
- Figured out the error from the end of yesterday where the blog component wasn’t rendering in the multiroute site. It’s because the NAMED import of your reducer gets added to the store by the same name. So if you add the `blog` reducer to the store, then your component’s mapStateToProps should have `articles: state.blog.articles`. That `blog` string must match. It wasn’t because the db was empty, the state.home object was truly undefined (bc it was state.blog instead).
- Added the scss back (scss = sassy css which is a different preprocessor than sass but basically the same intent).
- Deleted the blog repo from github (it was merged to my main portfolio.
- Installed bootstrap and its two reqs, jquery and popper, into node modules as deps of the project rather than from CDNs in the root index.html. Then all you have to do is `import ‘bootstrap/dist/css/bootstrap.css’;` if your main index.js. If you want to customize, you can add those scss files and import them below. https://facebook.github.io/create-react-app/docs/adding-bootstrap.
- Then I uninstall bootstrap popper and jquery and instead used react-bootstrap. It is all (most) of the pieces of bootstrap rewritten as react components. It doesn’t need jquery or any of that.
- Then I decided to go back to native bootstrap. I didn’t like how react-bootstrap forced you to write style and layout and such in react-specific syntax. If you ever switch the view library, it’s more work. You don’t have html and css and standard bootstrap syntax, you have react-specific bootstrap syntax.
- If you want the “active” capability, you also can’t go directly through react-bootstrap. That state is held by react-router, so you’re better off just using NavLink from that package.
- Applied both the default class and active class to the NavLink component itself, rather than surrounding it with a <button> or an <a> tag. This made it much cleaner. I’m getting better.
- Cleaned the whole oven interior from the jerky drippings months ago. Terrible chore, but the smoke output is much less now. I’ll never make the mistake again of not covering the bottom or putting a collection tray on the lower rack.
- The public/manifest.json file is just to describe metadata about the web app (author, homepage, etc). It’s like some of the content in setup.py. It’s where a homescreen shortcut would pull data from on a mobile phone, for an example API that manifest.json hooks into.
- NPM
- Two cool specifications for versions constraints.
- ^ means that it will take any minor version and patch version but will wait on major versions. For example, ^1.2.6 means that it will take 1.3 or 1.8.9, but will wait on 2.0.
- ~ is similar, but only for patch versions. It’s more restrictive. It will not autoupdate to any major or minor version.
- Installed the new 6.10.3 patch version of npm.
- To regenerate package-lock.json based on updates to package.json or other, just run `npm i –package-lock`.
- npm uninstall <pkg> to remove it from package.json and the lockfile.
- Github detected a few vulnerabilities in dep versions again: open, mime, braces, eslint, js-yaml, webpack-dev-server. This is such a cool feature. Ran the bot to attempt to autofix the minpins. Dependabot couldn’t resolve, so I explored a bit manually: `npm audit fix [–force]`.
- The issues were all transitive through an old version of react-scripts that I had pinned from an old tutorial. It was <1, whereas the most recent major version is 3.X.X.
- Nice, bmanning@co’s website for the new company: http://www.xonaspace.com/. Quick wordpress. No encryption tho.
- Reread a bit of the bootstrap docs for layout. Remember the grid system: container, row, cols.
- For cols, the numbers add up to 12 bc it’s divisible in multiple ways. You could have 2 equal width columns col-6 and col-6, or col-2 col-4 col-6 for 3 columns of increasing width.
- The letters xs sm md lg are for what size screen (device) you want these to apply. Mobile vs monitor etc.
- “col-lg-6 offset-lg-3” would be a cell that is half the screen width, offset to be centered (3-6-3).
- Bootstrap also has cards (used for my vertical blog posts), card-body and card-footer etc.
- Got the 3 mobiles apps for the flight legs, in order: united -> hawaiian -> american.
- Remember, my meal prices summarized: breakfast shake $2, lunch smoothie $4, veggie juice $3, dinner meat $3. Total daily ~$12.
- Downloaded Eloquent Javascript and Data Structures and Algorithms Made Easy as kindle books for Hawaii and the flights.
- Jest is a testing framework for js, just like jasmine. Jest can do more. Jasmine doesn’t use a dom so it doesn’t need a browser. It’s great for node development, because it only needs javascript. Jest, on the other hand, is more comprehensive. It does use a dom. It’s more popular.
- @myorg/mypkg is for scoping npm installs. It means that the package is owned by a certani group. This can be used for companies, or for open source things like @types/node. Then it will install all the package suffixes into node_modules/@types/*.
- Typescript.
- I’m not using babel/webpack directly, since I started with create-react-app. It uses react-scripts instead, which uses the js api for webpack instead of you using its cli directly in your build scripts.
- All I had to do was npm install typescripts and the many @types/*, then rename my js/jsx to ts/tsx.
- find . -name “*.jsx” -not -path “./node_modules/*” -not -path “./build/*” -not -path “*.json*” -exec bash -c ‘mv “$1” “${1%.jsx}”.tsx’ – ‘{}’ ;
- And the same with js/ts.
- Then restart the client app (npm start).
- src/index.ts has the main ReactDOM.render(<…>) syntax, so I had to rename it to tsx so it would compile. Without the x, that is (of course) not valid javascript/typescript.
- The @types/* libraries contain *.d.ts files, which are declarations for types.
- React passes a lot of ANY types around implicitly, like for props that can be flexible. It’s better to be explicit about all of that and predeclare what objects your props are, but for now I’m just specifying them as (props: any).
- There are vim plugins for typescript so that you get the desired syntax highlighting.
- Ended up not fully implementing this; reverted back to js. I got my hands dirty enough for basic familiarity.
-
- Added routing to my portfolio site, one for the current bouncing logos and one for the separate mern blog that I had built. Used react router v4, which is dynamic.
- https://codeburst.io/getting-started-with-react-router-5c978f70df91
- https://github.com/brianmahlstedt/bmahlstedt.com/issues/3
- All of the magic for npm start/build/eject/etc with create-react-app comes from the other package react-scripts. This is the same as flask-script.
- Added basic routing (react-router-dom.Router).
- Then added navigation links to the available routes (react-router-dom.Link).
- Then added 404 not found for routes that don’t exist (react-router-dom.Switch).
- Then added url parameters (/user/:id in the route, which comes up in this.props.match.params.id in the component).
- You can add nested routes, where one component is rendered in one route but then you load a subcomponent within that calls another route. I implemented this for practice, but then removed it because each of my SPA routes are mutually exclusive, but then added it back for practice again.
- Changed Link to NavLink, where you just add an activeClassName css class that defines how to style the current link.
- Remember the `exact` keyword for some react elements so that the route must strictly match in order to apply. Otherwise, /hello would try to apply both / and /hello because it technically matches both routes.
- Automatic navigation can occur on events as well. This is pretty easy. For example, if you submit a form, you can have it go back to the home page simply by onSubmit = this.props.history.push(‘/’)
- Merged the separate blog and bmahlstedt.com apps, repos, and functionalities. It’s now a single app with multiple routes; a home page, a logo page, and a blog page.
- I think that react-scripts assumes the entry point is src/index.js. I changed some component names around and npm start still worked. This is probably customizable, but I’ll leave it for now.
- Consumption of red meat has been linked to increased risk of colon cancer. Not common in my family history, but 1 instance.
- Sesame seeds grow into the sesame plant! It’s a little green shrub, as you’d expect, but it’s a bit strange to think of anything beyond the seed.
- Cacao powder is raw cacao bean (with fat removed and made into butter). Cocoa powder is roasted. You want the former, for its rawness.
- BOA deposit from ally cleared, moved a decent amount of it over to robinhood. That transfer can take up to 6 days, but it usually completes faster.
- You already know cross origin resource sharing (cors). Common use case: ajax to another domain. Other use: specifying when and where your assets can be accessed. Privacy.
- The browser makes the request, so the browser (not your service) first checks if it is going to another origin than the current site. If that other origin is not whitelisted with cors, the browser will reject it.
- Don’t store images in databases. They’re not built for it, and it can get complicated quickly. Most would suggest storing filepaths in the database that point to the respective images, so that you retain querying functionality etc.
- A grounding reminder that anxiety isn’t real: https://blog.heartsupport.com/anxiety-is-nothing-more-than-a-fear-of-what-if-7677d3b5f508. It’s just your brain extrapolating to the worst case scenario. “What if?” How about … no?
- react-cookie is the package that makes it very easy to add cookies to your components/routes.
- 20 minutes daily of stim and traction are well worth it. My elbow and neck are feeling better.
- Remember TENS is for nerve stimulation (pain relief) whereas EMS is for direct muscle stim (contracting for muscle development).
- Read a little bit about smoking a whole pig in preparation for the imu.
- A 100lb hog takes about 12 hours.
- Main yield: ribs, shoulders (butt/pulledpork), loin/tenderloin, and ham (legs).
- 250 is a good temp (lower is better, since you have different cuts and want equalization, but too low and it takes foreverrrr).
- Edible meat is about 40% of the original weight.
- The hog is gutted to the ribcage. It’s not spatchcocked, but it is opened and laid flat in a similar manner.
- Dry brine with salt before, then wet brine with olive oil and/or mustard. You want crackly skin. Rub the inside of the ribcage as well.
- Overall, not too different from my smaller sessions. The biggest complexity: cutting.
- Looked up the process for replacing the bent shift fork on the ninja’s second gear. It’s only slightly more than replacing the clutch plates. You drain the oil, open it up, remove the transmission assembly, then replace the shift fork. Each shift fork is $86.
- Pastrami and corned beef are both just cured for a week in a wet brine before cooking. You can add spices (juniper, mustard, celery, coriander, etc) or just use salt for the brine. The difference is that pastrami is usually smoked after curing, and corned beef is usually boiled. Another difference is that pastrami is usually a fattier beef cut (like the point) whereas corned beef usually starts with a leaner cut (like the flat).
- You can technically cure and smoke anything else – you could make pastrami beef ribs or pastrami pulled pork. It’s most common with beef cuts, but you can obviously cure any cut of meat in a wet spiced brine.
- style=”visibility:hidden;”
- Remember, chrome devtools has the react extension which gives you the full virtual dom, state/props for all components, and the ability to profile.
- Git won’t just stop tracking a file if it’s already staged, even if you added it to gitignore. You need to run git rm -r –cached <path>
- Updated and relaunched chrome.
- Good airbnb style guide for react: https://github.com/airbnb/javascript/tree/master/react.
- Use .jsx extension for files containing react components. Just use js for others. It doesn’t formally matter (the compiler takes care of it anyway), but it allows for separation of files in eslint targets, and stuff like that.
-
- MERN.
- If the backend uses newlines and the frontend uses breaks (almost always the case), you don’t need to do any casting. Just have the browser interpret it properly with `white-space: pre-line;`.
- It goes without saying, but the mongo db persists across both server and client app restart.
- Last quarter, netflix posted its first subscriber decrease in 8 years.
- Pre-fork worker process model is exactly as it sounds. Before a request comes in, the web server will pre-fork the master process into many.
-
- Typescript is awesome (as are the typing features of py3). I continue to save time as I convert.
- Closures are just the nomenclature for something most programmers already do. You have an outer function that defines some variables, defines an inner function that uses the variables, then returns the inner function for use. In this way, the actual function keeps a set of variables in scope however you choose. This can be for privacy, persistence, whatever. Example:
- outer = function() {
- var a = 1;
- var inner = function () {
- }
- return inner;
- }
- Coffee beans outgas too, just like I noticed on my sesame seed bags. That’s why they put those one-way valves in the coffee bags.
- I’m wary of bookmarking financial sites. If anybody gets the google creds, they can get a lot. However, if they get those creds, they can get a lot anyway (email, amazon, phone, etc).
- UFC on the east coast, which is awesome because the prelims start in the MORNING here.
- The current administration is taking some misinformed stances on encryption; that it’s scary, crime-enabling, etc. The privacy and security of 99% of the world’s communication is more valuable than the exposure of 1% of criminal texting. Here is a similar argument to show its fallacy: we should ban doors on homes because they enable criminals to elude law enforcement.
- LA’s population is over 10 million.
- Articles like this are so sadly off-target: https://medium.com/s/for-the-record/i-do-not-know-how-to-trust-thin-people-fbe78e633a50. Spreading this false lens is not good. You could rewrite the entire article in a light that doesn’t tarnish body positivity, switching a single word, and perhaps it would be more clear to those that believe the post:
- “I get drunk every day. I do not trust people who drink alcohol once a week, once a month, never, or any frequency less than mine. I indulge in it because it feels good, temporarily. I drink the next day again to persist the endorphin rush. Others do it less, and I acknowledge that. But I can’t respect it. I know this disposition might be bad for my health, but the short-term satisfaction is important to me. There are other explanations, of course. I’m predisposed to like the rush. I’m dealing with stress, and the seratonin helps me cope. My mind has a propensity for the addictive nature. I was born with a body that enjoys the stimulation more than most. But that’s ok. I’m happy with myself. I know that this pattern affects me physically. It affects others, as my behavior changes. I wear the consequences of my routines visibly on my body: the bags under my eyes, the smell, the hands clutched to my headache. Others can notice my habit, and they judge, but I can’t trust them. They’re biased. They don’t do it as often. They indulge less, so they can’t understand. They’re not concerned for me, they’re just condescending. They suddenly turn into public health authorities, thinking they know anything about my situation. Friends judge. Strangers stare. Even doctors. But the science is not clear. And they obviously don’t care about me, they just see my body as a walking manifestation of this so-called self destructive habit. I can’t trust people who refuse to get drunk every day.”
- There are no tigers in africa?
- Worked a lot on the MERN stack again, building my portfolio site.
- Read a little bit of the react-router and react-router-dom docs. I’m no expert yet, but the full picture is slowly becoming more clear.
- Removed title and author from the blog post components. Don’t need em.
- Just some easy reminders, coming from more familiarity with pip syntax. npm init to enter a wizard that adds a package.json to your cwd. npm i to install. Add -S to save it as a dep, which means adding it to package.json. Add -D to save it as a development dependency. When you npm i in a fresh clone with the package.json, it will create a node_modules folder and install all your deps locally into it.
- When you install core, it prints “Also, the author of core-js ( https://github.com/zloirock ) is looking for a good job -)” – yikes. Ads in packages. At the CLI. Nice UX…
- Got the client and server apps both working again, cleaned the repo, committed.
- Here is my diet, every single day:
- Breakfast shake: assorted powders (~$1), homemade oat milk ($1)
- Lunch smoothie: kale ($1.79), tomatoes ($0.79), banana ($0.30), turmeric ($0.20), ginger ($0.10), beet stalks (counted for beets instead)
- Preworkout juice: carrots ($0.75), red cabbage ($0.75), celery ($0.40), beets ($0.75)
- Dinner: almonds ($0.40), smoked meat ($2.00)
- Sometimes I’ll have a protein bar at the gym, which is $1.
- The veggies are organic, so the prices are a little bit higher than they would be otherwise.
- Powders: cinnamon, whey protein, creatine, cacao nibs, glutamine, lion’s mane, maca, flax.
- Other than that, it’s just coffee and water all day.
- Some of these are variable. Chicken vs beef, almonds vs seeds, etc, but the averages are close. Sometimes I’ll do sweet potatoes for breakfast, yogurt, bell pepper snack, etc. It washes.
- This obviously is just recurring consumable costs, not single purchase items like blender or infrastructural recurrence like charcoal, spices, etc.
- Rounding up, that’s $12/day to eat extremely healthily.

-
- The Ally transfer processed. I don’t see it in my BOA account yet, but I should get a large deposit notification soon. I also had $0.02 left in my Ally account so I just submitted a req for that as well.
- Went through some practice interview questions for js-specific roles (which I’m not applying to). I could answer about half, which I’m satisfied with. After studying, I’ll be able to get most. In any case, I’m seeking a full stack position.
- Read the tesla 2019Q2 report: https://ir.tesla.com/static-files/1e70a30c-20a7-48b3-a1f6-696a7c517959.
- Capex = capital expenditure (like overhead). Profit = revenue – capex.
- GAAP = generally accepted accounting principles.
- UrthBox was firm on “no” when I requested that they cancel the mistaken order (3mo subscription renewal), which was already shady. I pretended to enjoy their business, threatening the loss of future orders, making the decision to refund me lot easier for them. The agent spoke with his manager and magically found a way to cancel 2/3 of the order. This is a good strategy for dealing with cancellations/fees/etc. Paint the picture as a financial long-term strategy for them, rather than something subjective or political.
- Make sure to thank them after, even if you don’t plan on continuing being a customer of theirs. Pay it forward, ensure this model continues working. If you ghost them, the threats lose value on the macroscale over time.
- app.use() requires a middleware function. You do this for express.Router(), but not for something like your models. You just require() those (import them, don’t add them to the express app).
- 204 = no content. You might get some pending requests on an empty db.
- Always forget. lsb_release. Then use -h, or directly show version with -d.
- MERN.
- One of the reason my devtools network request was not responding (and I couldn’t find logs) was because the service wasn’t started (heh). I had begun the backend app days ago and had not restarted it once I had finished with the frontend. nodemon app.js.
- Moving on to the redux and state portion.
- Wrapped the app component in both a router and a redux provider, which has a store that contains reducers. Then you basically tell it that the state is all the articles, have it connect to the db, map the redux state to react props, then display the props (articles, blog posts) in the react component.
- There was another part completely missing from the tutorial lol: https://docs.mongodb.com/manual/tutorial/install-mongodb-on-ubuntu/. You need to have mongo both installed and running before it can obviously serve a localhost db, otherwise no data is being saved. Surprisingly …….. no errors from express when starting the app and trying to connect. At least not directly presented to the user in the cli. Yikes.
- Yup, that was totally why everything was busted. As I suspected above, the hanging requests were due to the backend waiting for db connections that … didn’t exist because there was no db. 1000% times better now, fast, clearer logs, everything.
- Finished the tutorial, got the baseline working. Now on to customization for my own purposes.
- Created a proper git repo for the blog and customized it.
- Remember, onsubmit is an event, the js function that executes when a form is submitted. onload is the same for when the page has loaded.
- … in js is the spread operator. If you have an object with multiple properties, and you want to create a second object with 99% the same properties, just changing a few of them, define the second one with the spread operator on the first object. obj2 = {…obj1, changedProp: x}
- Mongo
- Add key, add apt source, apt-get mongodb-org, sudo service mongod start.
- Then remember, you can check logs with journalctl -u <servicename>, or look in /var/log
- 27017 is the default mongo port.
- Enter shell with `mongo`. Just type `help`. Pretty similar to psql and postgres in general. Don’t need to terminate statements like psql.
- In the shell, show dbs, use <db>, show collections, <db>.<collection>.find()
- use <db> the db.dropDatabase() to delete one.
- moment.js is a great library for datetime manipulation, such as converting db timestamps into something relative to now, like “17 minutes ago”.
- Backticks in javascript are template literals. You can execute code inside of them, to insert expressions (like variables, or math) into strings.
- If you’re running the webpack dev server, you’ll see “wds live reloading enabled” in the browser console.
- React: be careful when using arrays, and assign each element a unique key. This applies to situations where you might be mapping an arbitrary-length list from db.find(), and rendering a <div> row for each or something. React uses keys to make it more efficient about what changed and what needs re-rendering. For all child elements in a component that has a list, give each one a unique id. https://reactjs.org/docs/reconciliation.html#keys. This is usually pretty easy, since the raw data has a db id in most cases that you can just pass through.
- These tattoos made to look like patches are incredible: https://tattooblend.com/hyperrealistic-patch-tattoos-by-duda-lozano/. Would be so cool to get one of a mission patch. This is my official copyright on that idea.
- npm prune remove unnecessary packages.
- Went through all the redux docs and took notes (in a google doc). Makes much more sense in the context of the simple MERN app I just built.
- Remember the react tab of chrome devtools to help debug and should the whole react virtual dom and everything.
- In javascript, `new` will execute the constructor when creating an object.
-
- Final closure on my ally account, realized gain: 6k. Basis was 10k started over 10 years ago to practice a little bit of private investment at the end of high school, got 60% overall.
- The ach from ally is on hold until tomorrow.
- Urthbox charged me another $90 for another 3 month subscription of snacks delivered to Eric’s house. I never wanted this, it was a one-time gift. Their fine print is bullshit and I emailed their support team.
- cprofile is a good profiler for python. @profile is a built-in decorator for memory profiling.
- numpy.ndarray can only hold one datatype, so it’s mucccccch more efficient than the generic python list (which has to allocate for flexible data types in every element).
- index.js are kinda like __init__.py files in python, spread through the project dirs to organize code and import/export availability within subpackages.
- In js, you can specify one and only one export as “default”, which means that imports can call it namelessly. Otherwise, you name your exports and import them by name.
- form-control is bootstrap. my-3 is a spacing example for bootstrap, which sets margin top and bottom (y-axis) to 3.
- Dayum. Finasteride, propecia, antibaldness drug can can impotence and anxiety: https://elemental.medium.com/my-life-has-been-ruined-by-an-anti-baldness-propecia-finasteride-side-effects-ed8b2fcd1e90.
- Police have had facial recognition database for a while. They can identify from mug shots, videos of crimes, etc. https://onezero.medium.com/california-police-are-sharing-facial-recognition-databases-to-id-suspects-3317726d31ad.
- While most are scared of technology like this, I’m a huge proponent. The more data, the better, even if private. This is an accountability problem, not a morality problem. 23andme genetic data, facial data, crime data, advertising data – take it all. I don’t have anything to hide, and data connections enable so many useful functionalities.
- MERN:
- High level index.js which does the ReactDOM.render().
- That imports components from other clean files, like a jsx that defines a Switch and a Route inside of a class or hook and exports it.
- AmEx anticipates vacations based on travel purchases, inferring that you’ll be away during certain dates and allowing transactions while there. Pretty cool, and not that hard.
- Placed fresh order.
- Morgan is the js library for request logging with node express apps.
- Axios is a library (wrapped around ajax using xml http requests) that allows you to make promise-based requests from the frontend or backend.
- Smoked chicken breast for softball. Tenderization is so important. I used a valentina bottle to pound them flat lol. Bought an actual tenderizer online. Going to try the 48 blade puncture tenderizer instead of the mallet variety.
- NFL is back!! Preaseason week 1 tonight, TNF, broncos vs falcons.
- Trichinella dies at 137F. Salmonella dies at a higher temp, and it takes a bit longer. Getting it above 160 is fine, 165 is even safer.